Best Practices: Write Unit Tests
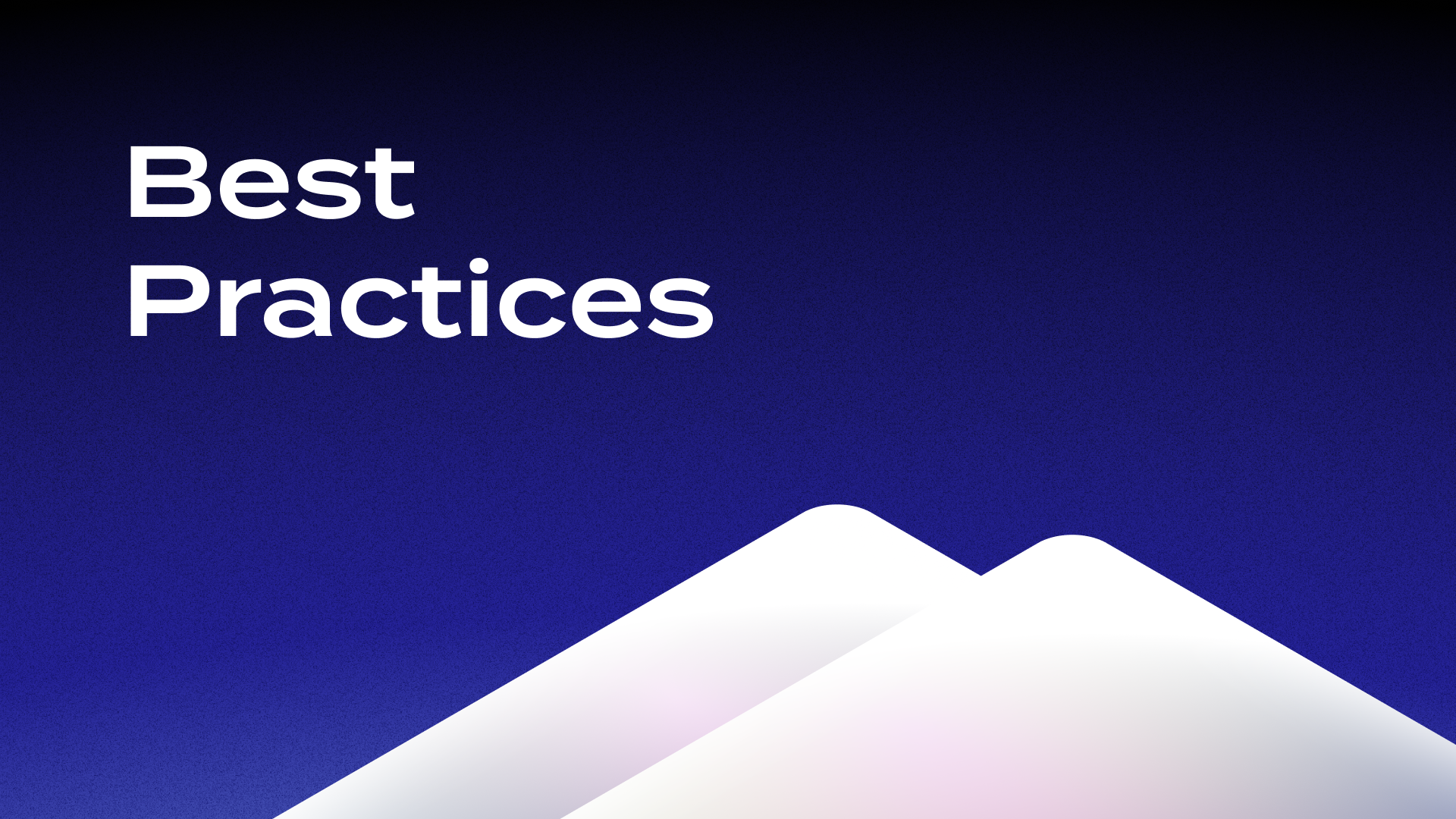
Introduction
Writing unit tests is a fundamental aspect of blockchain development, ensuring that each component of your application functions correctly. This chapter provides a practical guide on how to write unit tests, focusing on best practices and methodologies to enhance the reliability and maintainability of your blockchain application.
Step 1: Understand What to Test
Begin by identifying the key components of your blockchain application that require testing. In a typical blockchain module, these might include:
- Message Handlers: Test the logic for handling different types of messages.
- Keepers: Validate the logic for state management.
- Query Handlers: Ensure queries return the correct data.
Step 2: Setting Up the Test Environment
-
Create a Test File: For a Go module, a test file typically ends with
_test.go
. -
Import Necessary Packages: Include the testing package and any other dependencies required by your module.
import (
"testing"
"github.com/cosmos/cosmos-sdk/types"
)
Step 3: Write Test Functions
Each test function in Go starts with Test
followed by a descriptive name and takes a *testing.T
parameter.
func TestHandler_CreateItem(t *testing.T) {
// Test code here
}
Step 4: Initialize Test Inputs
-
Setup Mock Context: Use sdk.NewContext and other Cosmos SDK utilities to create a mock context.
-
Create Test Cases: Define various scenarios for your test, including edge cases and typical use cases.
tests := []struct {
name string
msg MsgCreateItem
expectErr bool
}{
{"valid case", NewMsgCreateItem(...), false},
{"invalid case", NewMsgCreateItem(...), true},
}
Step 5: Writing Test Logic
For each test case:
-
Call the Function: Call the function you're testing with the test input.
-
Check the Result: Use require or assert statements to validate the outcome.
for _, tc := range tests {
t.Run(tc.name, func(t *testing.T) {
_, err := handler(ctx, tc.msg)
if tc.expectErr {
require.Error(t, err)
} else {
require.NoError(t, err)
}
})
}
Step 6: Running Your Tests
Run your tests using the go test
command in your module's directory. You can run all tests or specify a particular test file
go test ./...
Step 7: Best Practices
- Clear and Understandable Tests: Write tests that are easy to read and understand.
- Cover Edge Cases: Include tests for exceptional and boundary conditions.
- Maintain Tests: Keep your tests up-to-date with code changes.
Conclusion
Unit testing is an indispensable part of the blockchain development lifecycle. It not only ensures code quality and functionality but also provides documentation and aids in future code modifications. By following these steps and best practices, you can create effective unit tests that bolster the reliability and security of your blockchain application.