Spotlight: Ignite/Cosmos Chain Version Control
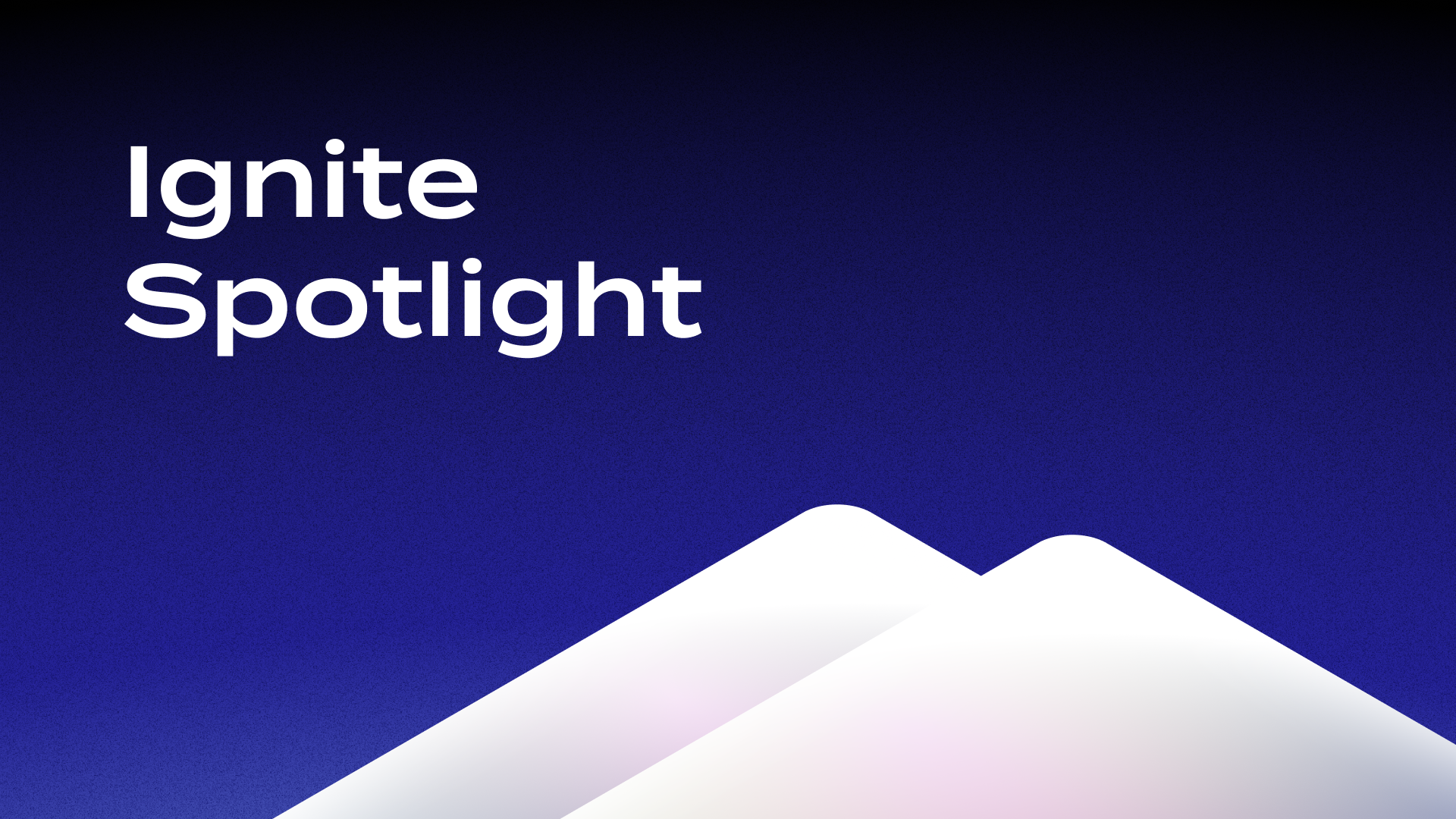
To effectively release and version your Ignite created Cosmos SDK chain software, ensuring the binary properly displays the version and users can easily fetch it from GitHub, you'll want to follow best practices around versioning and using tools like Ignite CLI. Here’s a streamlined process to achieve this:
1. Semantic Versioning
Adopt semantic versioning (SemVer), which uses a three-part version number: major, minor, and patch (e.g., v1.0.0). This helps communicate changes and stability in your updates:
- Major version changes indicate breaking changes.
- Minor version changes introduce new features but are backward compatible.
- Patch version changes are for backward-compatible bug fixes.
2. Use Git Tags
Using Git tags helps to mark a specific point in your repository's history as important, typically used for releases:
- Create a tag in Git to indicate a release:
git tag -a v1.0.0 -m "Release version 1.0.0"
- Push the tags to GitHub:
git push origin --tags
Good Practices
- Document everything: Make sure that each release has clear and detailed release notes explaining the changes.
- Automate processes: Use CI/CD pipelines to automate testing, building, and deploying your software.
- Communicate with your community: Keep the users of your software in the loop about upcoming changes, deprecations, and improvements.
3. Consensus-Breaking Changes in the Cosmos SDK
Consensus-breaking changes occur when updates to the blockchain software modify the rules of transaction validation or the blockchain state in a way that is not compatible with earlier versions. These changes require all validators to upgrade to the new version to maintain consensus and avoid network forks.
Key Steps for Managing Consensus-Breaking Changes
- Identify the Changes: Clearly identify and document the changes that will break consensus. This includes changes to the transaction structure, state machine, consensus rules, or any other component that affects how blocks are validated.
- Versioning: Consensus-breaking changes should increment the major version number as per Semantic Versioning (e.g., from
1.x.x
to2.0.0
). This indicates a breaking change that is not backward compatible. - On-Chain Governance:
- Upgrade Proposal: Propose an on-chain governance proposal for the upgrade. This proposal should detail the changes, reasons, impacts, and the proposed activation block height.
- Voting: Allow validators and stakeholders to vote on the proposal. A successful vote means that there is consensus to implement the changes.
- Scheduling: If approved, the upgrade is scheduled for a specific block height at which the new rules will come into effect.
- Upgrade Handlers: Implement upgrade handlers in your Cosmos SDK application to handle the state migration or any necessary transformations to the new software version.
-
Registering an Upgrade Handler: Use the
app.UpgradeKeeper
module to manage the software upgrades. Here’s a basic example of how to set this up:import ( "github.com/cosmos/cosmos-sdk/x/upgrade" ) func RegisterUpgradeHandlers(app *baseapp.BaseApp) { app.UpgradeKeeper.SetUpgradeHandler("v2.0.0", func(ctx sdk.Context, plan upgrade.Plan) { // Logic for transitioning from v1.x.x to v2.0.0 }) }
Read
zeroFruit
's Blogpost to learn more about implementing upgrade handlers and migrating Modules: https://medium.com/web3-surfers/cosmos-dev-series-cosmos-sdk-based-blockchain-upgrade-b5e99181554c
-
Handling Non-Breaking Changes
Non-breaking changes, such as minor feature enhancements or bug fixes, do not require coordination via on-chain governance and can be handled through minor or patch version upgrades. These updates should still be tested thoroughly but do not necessitate a network-wide coordinated upgrade.
Best Practices
- Testing: Rigorously test all updates, especially consensus-breaking changes, on testnets under conditions as close as possible to the mainnet environment.
- Communication: Maintain clear and open communication channels with all network participants about upcoming changes, requirements, and timelines for upgrades.
- Documentation: Provide detailed release notes and upgrade guides to assist validators and other stakeholders in understanding the changes and the steps required to implement the upgrade.
Conclusion
Handling consensus-breaking changes with the Cosmos SDK involves careful planning, clear communication, and rigorous testing to ensure network stability and continuity. By using the built-in upgrade modules of the Cosmos SDK and following best practices, you can manage these changes effectively and maintain the trust and reliability of your blockchain network.
For further details and advanced scenarios, the Cosmos SDK documentation and community forums are invaluable resources for support and guidance.