Best Practices: Writing Effective Unit Tests in Blockchain Development
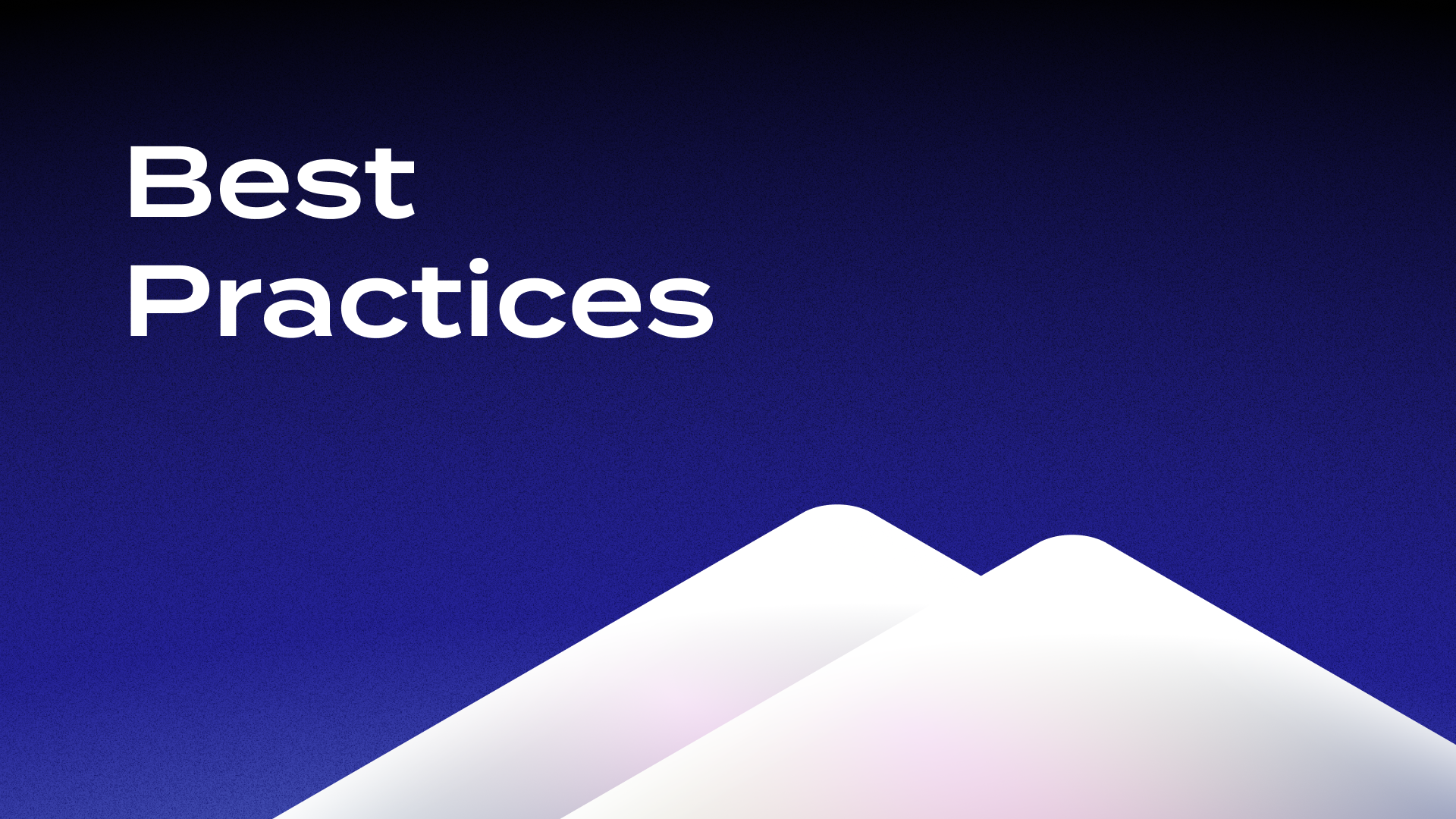
Introduction
Unit testing is a vital part of blockchain development, ensuring that individual components of your application work as expected. Ignite CLI, with its integration into the Cosmos SDK ecosystem, offers a streamlined approach to writing and managing unit tests. This chapter will guide you through best practices for leveraging Ignite CLI to create effective unit tests for your blockchain applications.
Step 1: Understanding the Cosmos SDK Testing Framework
Before diving into writing tests, it's important to understand the Cosmos SDK testing framework. Cosmos SDK provides a comprehensive set of tools and libraries for testing modules and handlers. It includes:
simapp
: A simulated app environment for testing modules.keeper
test helpers: For setting up keepers with necessary dependencies.- Mocking frameworks: To create mock objects and simulate real-world scenarios.
Step 2: Setting Up Your Testing Environment
After scaffolding your blockchain with Ignite CLI, you'll find a test directory in each module. This is where your unit tests will reside. Ensure you have a testing environment set up with:
- Go testing libraries.
- Cosmos SDK's testing utilities.
- Any additional mocking libraries you might use (e.g., gomock, testify).
Step 3: Writing Unit Tests for Modules
When writing unit tests for modules:
- Test Initialization: Ensure each test begins with a clean state. Use the
simapp
to initialize modules and keepers.
app := simapp.Setup(false)
ctx := app.BaseApp.NewContext(false, tmproto.Header{})
- Keepers and Handlers: Test keepers and handlers separately. For keepers, focus on state changes and storage. For handlers, concentrate on the processing of messages and validation.
// Example keeper test
require.Equal(t, expectedValue, keeper.GetYourValue(ctx))
// Example handler test
_, err := handler(ctx, &types.MsgYourType{})
require.NoError(t, err)
- Mocking Dependencies: Use mocking frameworks to simulate external dependencies and focus tests on the component being tested.
Step 4: Test-Driven Development (TDD)
Embrace TDD for robust development:
- Write Tests First: Define how components should behave.
- Implement Features: Write code to pass the tests.
- Refactor: Improve code while ensuring tests still pass.
Step 5: Integrating Tests into Your Workflow
- Continuous Integration (CI): Automate running tests with each commit using GitHub Actions or other CI tools.
- Code Reviews: Include passing tests as a requirement for merging pull requests.
Step 6: Running and Maintaining Tests
- Run Tests Regularly: Use go test ./... in your module directory to run all tests.
- Keep Tests Updated: Update tests in tandem with feature changes.
Step 7: Best Practices and Tips
- Clear and Concise Tests: Write tests that are easy to understand and maintain.
- Edge Cases: Test edge cases and error conditions.
- Documentation: Document your tests for better maintainability.
Step 8: Using Ignite CLI for Testing
While Ignite CLI doesn't directly scaffold tests, it sets up a structure conducive to effective testing. Familiarize yourself with the generated code structure and where to place your tests.
Conclusion
Effective unit testing is critical in blockchain development for ensuring reliability and security. By following these guidelines and integrating Ignite CLI’s capabilities, you can create a robust testing environment that contributes significantly to the quality of your blockchain applications.